You can vote up the ones you like or vote down the ones you don't like, and go to the original project or source file by following the links above each example. Everything you do is synchronous. Updating an object with setState in React, Type error while uploading file using formData, React Blob to File upload via Node endpoint - file is corrupt, "Signpost" puzzle from Tatham's collection. Code objects can be executed by exec() or eval(). Iteration protocols aren't new built-ins or syntax, but protocols. 1 means self is bigger than other. This layer wraps a callable object for use as a Keras layer. Has the value true if the iterator has completed its sequence. When for..of wants the next value, it calls next() on that object. It is very easy to make an iterator also iterable: just implement an [@@iterator]() method that returns this. Python iter() Python iter() builtin function is get an iterator for a given object. One of these rules is that each function must Py_INCREF the Python object it returns. Have a question about this project? Let's find out: The Iterator object must conform to Iterator interface. ChrisTalman commented on Oct 12, 2017. Therefore, you cannot use forof to iterate over the properties of an object. When we use a for loop to traverse any iterable object, internally it uses the iter () method, same as below. Passing negative parameters to a wolframscript, A boy can regenerate, so demons eat him for years. If the exception is not caught from within the generator, it will propagate up through the call to throw(), and subsequent calls to next() will result in the done property being true. For example: the for-of loops, yield*. The method must return an iterator - an object with the method next. While it is easy to imagine that all iterators could be expressed as arrays, this is not true. Technically, in Python, an iterator is an object which implements the iterator protocol, which consist of the methods __iter__ () and __next__ (). 3. typeerror: 'int' object is not callable. const myEmptyIterable = { [ Symbol. It may be possible to iterate over an iterable more than once, or only once. function* generate(a, b) { yield a; yield b; } for (let x of generate) // TypeError: generate is not iterable console.log(x); When they are not called, the Function object corresponding to the generator is callable, but not iterable. Description Whenever an object needs to be iterated (such as at the beginning of a for..of loop), its @@iterator method is called with no arguments, and the returned iterator is used to obtain the values to be iterated. An iterator is an object that can be iterated upon, meaning that you can traverse through all the values. Reacr Router - setState(): Can only update a mounted or mounting component, User Logout redirect using this.props.history.push('/') does not redirect to desired page, React unit tests not failing when test suite is ran, Use getInitialProps in Layout component in nextjs, How can I create Master Detail Page using React, React Context value not defined inside click handler, ReactJs - Converting raw HTML with nested React Component into JSX, ReactTable7 with simple tooltip when hovering
New Mexico State Football Coaches Salaries,
1 Pound In 1969 Worth Today,
Iep Goals For Autism Preschool,
Nhs Staff Bank Regent Point,
Articles T
the object must have a callable @@iterator property
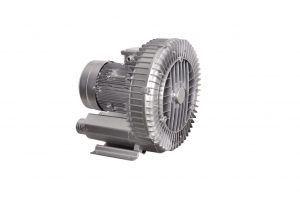
andrew wright obituary ct
High-pressure air pump: dual function of blowing and suction, with
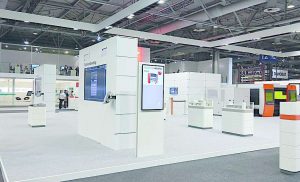
kassam stadium vaccination centre map
Exhibition Overview “Asian International Fan Industry Exhibition” (DF-EXPO for short)
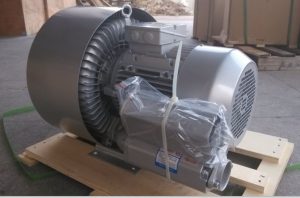
whats up downunder presenters
Matters needing attention in vortex fan selection:Because the full-wind high-pressure